If you don't know how to save images into the database then you can read my recent post that i've made "Saving Images in a Database in an asp.net Website."
Click here for more details.
I am using C# as programming language and Sql Server as a back end.
Now that we have images in our database, We can easily retrieve those images and show them in a Image control in the Asp.net Page.
So, Here is the Table schema that we're going to use in this exercise.
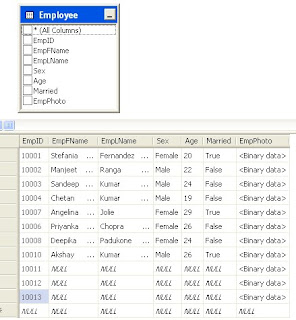
Drag a Datalist control on to the form.
Set Repeat property to Flow, RepeatDirection Property to Horizontal and RepeatColumns to 2.
The following is the markup of Default.aspx page.
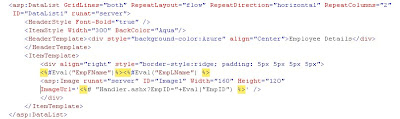
The following is the markup of Default.aspx.cs page.
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class _Default : System.Web.UI.Page
{
static string constring;
protected void Page_Load(object sender, EventArgs e)
{
constring = ConfigurationManager.ConnectionStrings["myConn"].ConnectionString;
if (!IsPostBack)
{
BindData();
}
}
protected void BindData()
{
using (SqlConnection con = new SqlConnection(constring))
{
con.Open();
SqlDataAdapter adap = new SqlDataAdapter("Select EmpFName,EmpLName,EmpPhoto,EmpID from employee", con);
DataSet ds = new DataSet();
adap.Fill(ds, "Employee");
DataList1.DataSource = ds.Tables["Employee"].DefaultView;
DataList1.DataBind();
}
}
Add a connection string tag to the web.config file so that we can retrive that connection information whereever we want.

Now as our images are in the database, and we want to show them in an .aspx page, We need a handler for our images who can handle the retrieval of images.
So, Add an handler.ashx to your website by clicking Website->AddNewItem and Selecting Generic Handler from the AddNewItem Dialog box. Name it as Handler.ashx
and type the following code in your handler.ashx file
using System;
using System.Web;
using System.Configuration;
using System.Data.SqlClient;
public class Handler : IHttpHandler
{
static string constring;
public void ProcessRequest (HttpContext context)
{
constring = ConfigurationManager.ConnectionStrings["myConn"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
SqlCommand cmd = new SqlCommand("Select EmpPhoto from Employee where EmpID=@EmpID", con);
cmd.Parameters.AddWithValue("EmpID", context.Request.QueryString["EmpID"].ToString());
con.Open();
SqlDataReader dr = cmd.ExecuteReader();
dr.Read();
context.Response.BinaryWrite((byte[])dr["EmpPhoto"]);
dr.Close();
}
}
public bool IsReusable
{
get
{
return false;
}
}
}
Now run your website, and You will see all the images in the DataList Like this.
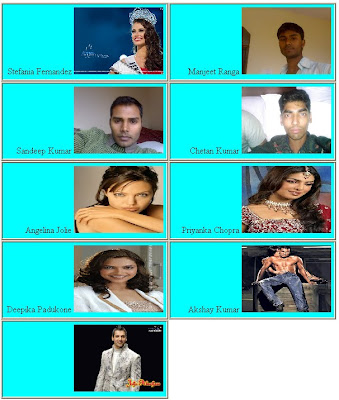