I am using C# as programming language and Sql Server as a back end.
First of all we need to create a datatable where we will be storing the images in the database.
Use any database where you want to create the datatable.
This is how the Datatable structure looks like:
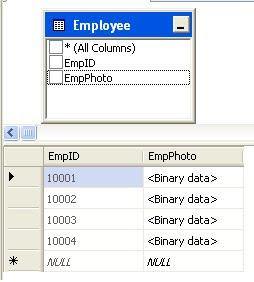
Now Create the table by typing:
CREATE TABLE [dbo].[Employee]
(
[EmpID] [int] IDENTITY(10001,1) NOT NULL,
[EmpPhoto] [image] NULL
)
Now, create a new Web Site the file menu->New Website option.
Then drag a FileUpload control on to the form.
Then drag a button on the form.
Then drag a Label on the form.
Change the Id of the Button to btnUpload and text to Upload.
Change the Id of the Label to lblMessage.
Double click on the Upload Button to generate the default event handler for browse button and type the following code into it.
protected void btnUpload_Click(object sender, EventArgs e)
{
if (FileUpload1.PostedFile.FileName != "" && FileUpload1.PostedFile != null)
{
HttpPostedFile file = FileUpload1.PostedFile;
byte[] size = new byte[file.ContentLength];
file.InputStream.Read(size, 0, (int)file.ContentLength);
using (SqlConnection con = new SqlConnection(constring))
{
SqlCommand cmd = new SqlCommand("insert Employee (EmpPhoto) values (@Photo)", con);
cmd.Parameters.Add("Photo", SqlDbType.VarBinary, size.Length).Value = size;
con.Open();
int result = cmd.ExecuteNonQuery();
if (result > 0)
{
lblMessage.Text = "File Uploaded";
}
else
{
lblMessage.Text = "File not uploaded";
}
con.Close();
cmd.Dispose();
con.Dispose();
}
}
}
Now that, you have done all the coding. Just Press F5 to run the Website
Click the browse button to browse a image file.
Now click the upload button to upload the image to the database.
Message will be shown to you stating that your image is uploaded successfully.
Now, We have images stored in the database. We can show them into the Image Control in our Website. We You want to show images in the Gridview, then read my post "Retrieving images from the database"
Click here for more details.
sir where is the connection string syntax and format............
ReplyDelete