I am using C# as programming language and Sql Server as a back end.
First of all we need to create a datatable where we will be storing the images in the database.
Use any database where you want to create the datatable.
This is how the Datatable structure looks like:
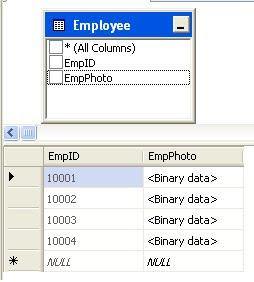
Now Create the table by typing:
CREATE TABLE [dbo].[Employee]
(
[EmpID] [int] IDENTITY(10001,1) NOT NULL,
[EmpPhoto] [image] NULL
)
This is how the form design should look like:
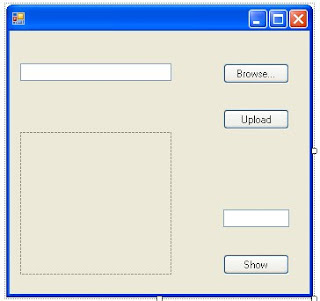
Now, create a new windows application from the file menu->New Projects option.
Then drag a Text dialog box on to the form.
Then drag two buttons on the form.
Name the first button btnBrowse and set its text property as Browse...
Name the Second button btnUpload and set its text property as Upload.
Name the textbox as txtPath
Now Import two namespaces i.e.
using System.Data.SqlClient;
using System.IO;
Double click on the Browse button to generate the default event handler for browse button and type the following code into it.
private void btnBrowse_Click(object sender, EventArgs e)
{
op = new OpenFileDialog();
op.ShowDialog();
txtPath.Text = op.FileName;
}
The above code will show an open file dialog and sets the text of the text box to the path of the file that you will select in the open file dialog box.
Now, Double click on the Upload button to generate the default event handler for Upload button and type the following code into it.
private void btnUpload_Click(object sender, EventArgs e)
{
byte[] image;
if (txtPath.Text != "")
{
string fileName = txtPath.Text;
using (FileStream fs = new FileStream(fileName, FileMode.Open))
{
BinaryReader reader = new BinaryReader(fs);
image = reader.ReadBytes((int)fs.Length);
fs.Close();
}
using (SqlConnection conn = new SqlConnection("data source=xyz;initial catalog=post;Integrated Security=True"))
{
SqlCommand command = new SqlCommand("INSERT INTO Employee (EmpPhoto) VALUES (@Photo)", conn);
command.Parameters.Add("@Photo", SqlDbType.Image).Value = image;
conn.Open();
command.ExecuteNonQuery();
}
MessageBox.Show("Image Uploaded Succesfully");
}
else
MessageBox.Show("Please select a file to upload");
}
Now that, you have done all the coding. Just Press F5 to run the project.
Click the browse button to browse a image file.
Now click the upload button to upload the image to the database.
Message will be shown to you stating that your image is uploaded successfully.
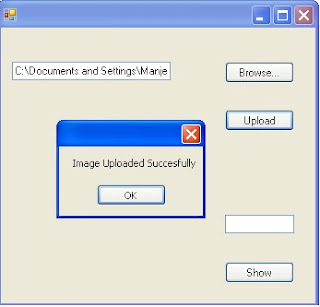
Now, We have images stored in the database. We can show them into the picture box in our application. If want to show images in the picture box, then read my post "Retrieving images from the database"
Click here for more details.
No comments:
Post a Comment